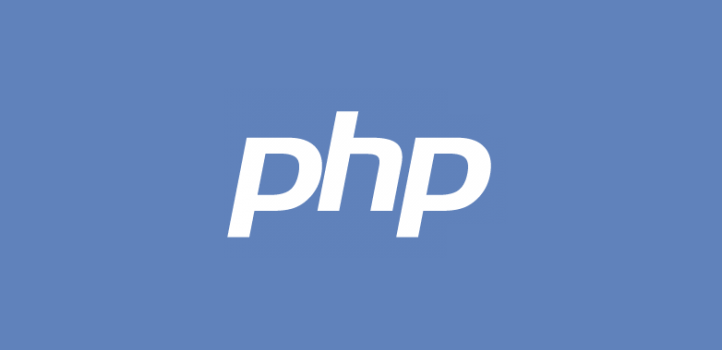
Tags:
I had a request recently to get the size of a large collection of files that were hosted on Amazons S3 storage and add the values to a database containing a list of these files that acted as a local cache. I saw two main ways to achieve this: mount the S3 storage and treat the files as if they were local, or query the files on the fly as the sizes are required.
I went for the second method, which was actually a lot easier, mostly due to the fact that the server this script was intended to run on didn't allow me the level of access I'd need to mount a remote file system.
The script itself is fairly simple; use cURL to make a HEAD request, echo the returned Content-Length
value, and that's it!
$remoteFile = 'http://ashleysheridan.co.uk/img/articles/canvas_glow.png';
$ch = curl_init($remoteFile);
curl_setopt($ch, CURLOPT_NOBODY, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HEADER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
$data = curl_exec($ch);
curl_close($ch);
$contentLength = 0;
if($data !== false)
{
if (preg_match('/Content-Length: (\d+)/', $data, $matches))
{
$contentLength = (int)$matches[1];
}
}
echo $contentLength;
Lines 3 through 6 set up the options for cURL, the last of which is only necessary if you are making a request from a site which uses redirects, like mine does to always force the www part on the url. The preg_match
line just pulls out the information from the header result that we want. If for some reason the file size wasn't available, then the script will just output a 0.
Comments